3 minutes
Setting up a Flutter Development Environment on Ubuntu, Manjaro, Arch Linux Without Installing Android Studio
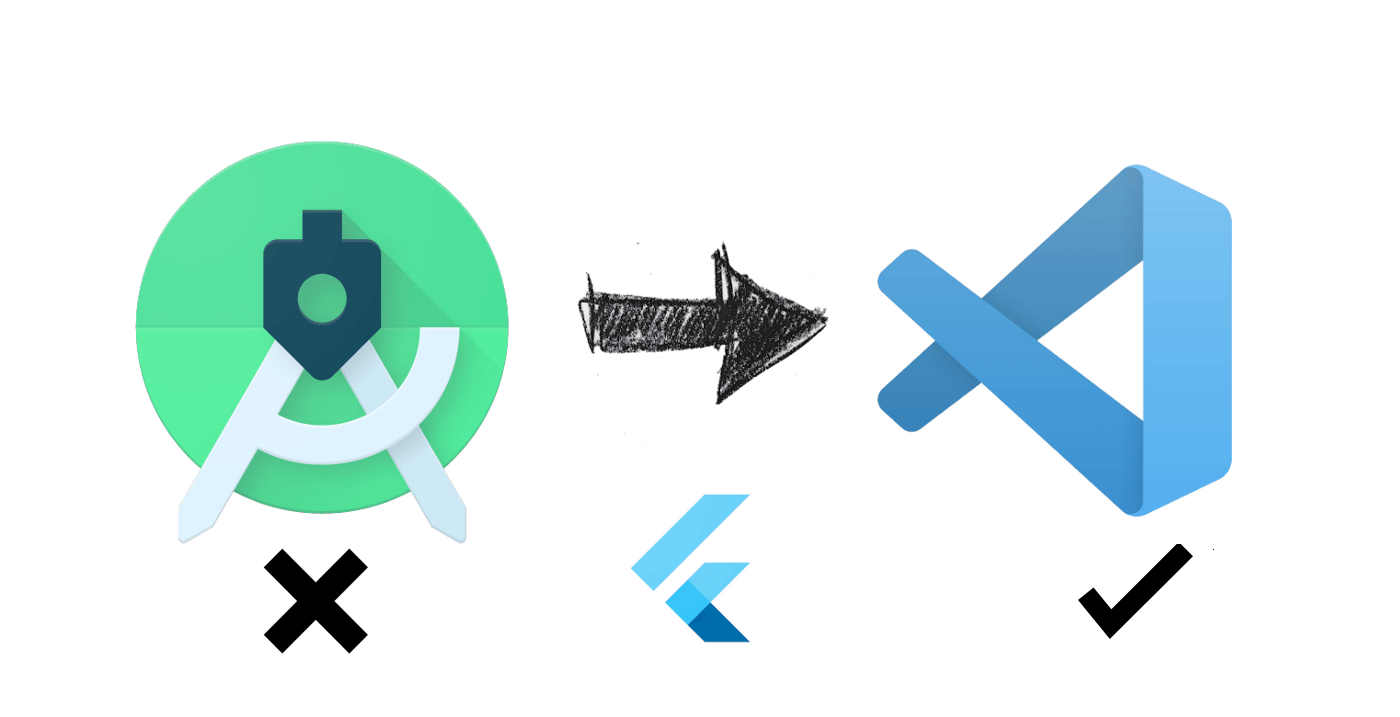
Setting up Flutter on Linux is pretty much straightforward. The official documentation is well detailed and numerous tutorials on the web explain how to configure a Flutter development environment on Linux. However, installing Android Studio is a major requirement as Flutter depends on several packages and libraries that ship with Android Studio.
A lot of people (myself included) prefer to use another editor such as Visual Studio Code instead of Android Studio to develop Flutter applications for various reasons, one of which is that VS Code is more lightweight than Android Studio. The main concern that follows is the “wasted” disk space due to Android Studio’s installation.
Being a minimalist, I decided to tinker around and after a few days of trial and error, I managed to get Flutter to work without installing or depending on Android Studio by writing some Bash scripts that automate the whole process.
Solution
Step 1: Install OpenJDK
Create a file install-jdk.sh
with the following contents:
#!/bin/bash
## File: install-jdk.sh
## Copyright © 2022 Muzaffar Rayyan Auhammud
## License: MIT License (https://mit-license.org/)
JDK_VERSION=18.0.1.1
JDK_ROOT=/opt/openjdk
JAVA_HOME=$JDK_ROOT/jdk-$JDK_VERSION
ENV_FILE="~/.profile"
DISTRO=$(uname -r)
if [[ $DISTRO == *"MANJARO"* || $DISTRO == *"ARCH"* ]]
then
ENV_FILE="/etc/profile"
fi
echo "" | sudo tee -a $ENV_FILE
cd ~/Downloads
wget -c https://download.java.net/java/GA/jdk${JDK_VERSION}/65ae32619e2f40f3a9af3af1851d6e19/2/GPL/openjdk-${JDK_VERSION}_linux-x64_bin.tar.gz
sudo mkdir -p $JDK_ROOT && sudo tar xzf openjdk-${JDK_VERSION}_linux-x64_bin.tar.gz -C $JDK_ROOT
echo "export JAVA_HOME=$JAVA_HOME" | sudo tee -a $ENV_FILE
echo "export PATH=\$PATH:\$JAVA_HOME/bin" | sudo tee -a $ENV_FILE
source $ENV_FILE
Open a Terminal window and run the script as follows:
bash install-jdk.sh
Step 2: Install Android SDK
Create a file install-android-sdk.sh
with the following contents:
#!/bin/bash
## File: install-android-sdk.sh
## Copyright © 2022 Muzaffar Rayyan Auhammud
## License: MIT License (https://mit-license.org/)
ENV_FILE="~/.profile"
DISTRO=$(uname -r)
if [[ $DISTRO == *"MANJARO"* || $DISTRO == *"ARCH"* ]]
then
ENV_FILE="/etc/profile"
fi
echo "" | sudo tee -a $ENV_FILE
ANDROID_HOME=$HOME/Android/Sdk
cd ~/Downloads && wget -c https://dl.google.com/android/repository/commandlinetools-linux-8512546_latest.zip
mkdir -p $ANDROID_HOME/cmdline-tools && unzip commandlinetools-linux-8512546_latest.zip -d $ANDROID_HOME/cmdline-tools
mv $ANDROID_HOME/cmdline-tools/cmdline-tools $ANDROID_HOME/cmdline-tools/latest
cd ~/Downloads && wget -c https://dl.google.com/android/repository/platform-tools-latest-linux.zip
mkdir -p $ANDROID_HOME && unzip platform-tools-latest-linux.zip -d $ANDROID_HOME
echo "export ANDROID_HOME=$ANDROID_HOME" | sudo tee -a $ENV_FILE
echo "export PATH=\$PATH:\$ANDROID_HOME/platform-tools" | sudo tee -a $ENV_FILE
echo "export PATH=\$PATH:\$ANDROID_HOME/cmdline-tools/latest/bin" | sudo tee -a $ENV_FILE
echo "export PATH=\$PATH:\$ANDROID_HOME/emulator" | sudo tee -a $ENV_FILE
source $ENV_FILE
# Android 10 Q Platform API and build tools
sdkmanager --install "platforms;android-31" "build-tools;30.0.3"
emulator -accel-check
Just like before, open a Terminal window and run the installation script as follows:
bash install-android-sdk.sh
Step 3: Install Flutter SDK
Create a file install-flutter-sdk.sh
with the following contents:
#!/bin/bash
## File: install-flutter-sdk.sh
## Copyright © 2022 Muzaffar Rayyan Auhammud
## License: MIT License (https://mit-license.org/)
ANDROID_HOME=$HOME/Android/Sdk
sudo snap install flutter --classic
flutter upgrade
flutter config --android-sdk $ANDROID_HOME
flutter doctor --android-licenses
flutter doctor
flutter config --no-analytics
dart --disable-analytics
Similar to the above steps, open a Terminal window and run the installation script as follows:
bash install-flutter-sdk.sh
Accept all the licenses when prompted, and you should have a complete Flutter development environment without requiring Android Studio to be installed.
Note
Then, run the following command in a Terminal window and grant your phone permission to access the computer through ADB when prompted:
sudo adb kill-server && \
sudo adb start-server && \
adb devices
Comments
Please wait...